Examination of the Code#
The following provides a brief explanation of the Inrupt’s JavaScript client libraries usage in the Getting Started application code.
Login Code#
The example uses Inrupt’s @inrupt/solid-client-authn-browser
library to log in.
@inrupt/solid-client-authn-browser
is for client-side code only.
For applications implementing Authorization Code Flow:
The application starts the login process by sending the user to the user’s Solid Identity Provider.
The user logs in to the Solid Identity Provider.
The Solid Identity Provider sends the user back to your application, where the application handles the returned authentication information to complete the login process.
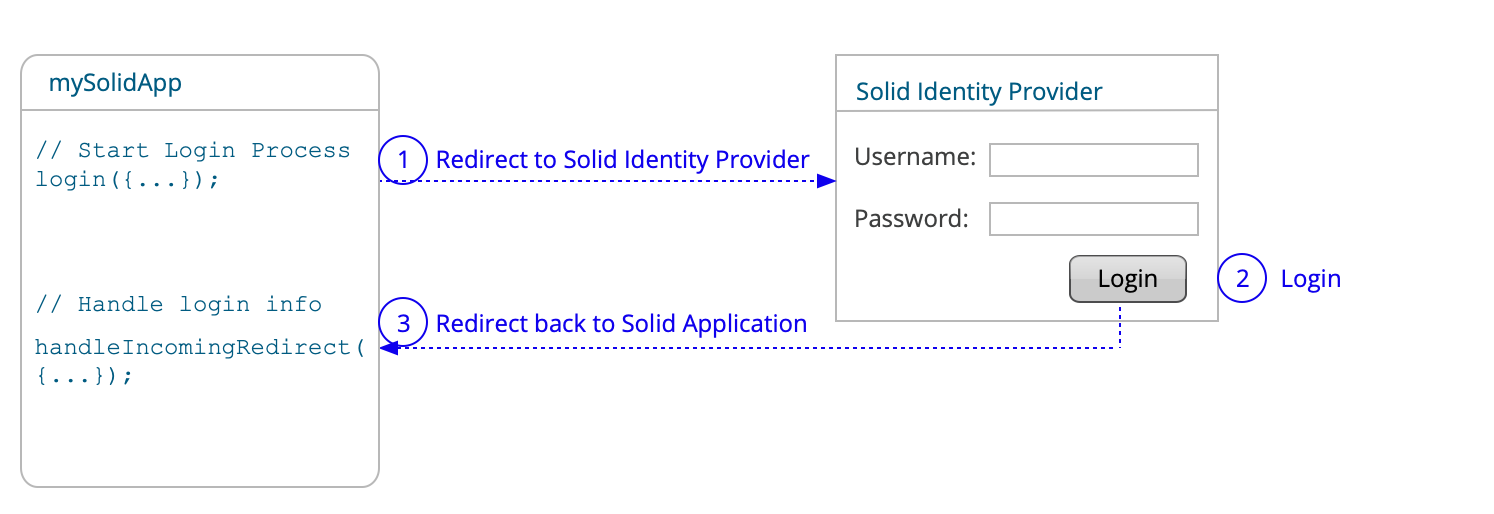
Import#
The application uses various objects from
@inrupt/solid-client-authn-browser
to log in.
import {
login,
handleIncomingRedirect,
getDefaultSession,
fetch
} from "@inrupt/solid-client-authn-browser";
Start Login#
The application starts the login process by calling login() with the following options:
|
The URL of the user’s Solid Identity Provider. The function sends the user to this URL to log in. In this example, it is set to the value selected by the user. Note If you are not using PodSpaces, modify
the |
|
The URL where the user, after logging in, will be redirected in order to finish the login process. Note The In this example, it is set to |
|
A user-friendly application name to be passed to the Solid Identity Provider. The value is displayed in the Identity Provider’s Access Request approval window. |
function loginToSelectedIdP() {
const SELECTED_IDP = document.getElementById("select-idp").value;
return login({
oidcIssuer: SELECTED_IDP,
redirectUrl: new URL("/", window.location.href).toString(),
clientName: "Getting started app"
});
}
The login() function
sends the user to the Solid Identity Provider specified in
oidcIssuer
. Once the user logs in with the Identity Provider, the user is
redirected back to the specified redirectUrl
to finish the
login process.
Finish Login#
Once logged in at the Solid Identity Provider, the user is redirected
back to the redirectUrl
specified at the start of the login
process (i.e., in the login()
function call). The
page at this redirectUrl
calls
handleIncomingRedirect() to complete the login process.
In the example, the page calls handleRedirectAfterLogin()
method,
which, in turn, calls the
handleIncomingRedirect():
// When redirected after login, finish the process by retrieving session information.
async function handleRedirectAfterLogin() {
await handleIncomingRedirect(); // no-op if not part of login redirect
const session = getDefaultSession();
if (session.info.isLoggedIn) {
// Update the page with the status.
document.getElementById("myWebID").value = session.info.webId;
// Enable Read button to read Pod URL
buttonRead.removeAttribute("disabled");
}
}
// The example has the login redirect back to the root page.
// The page calls this method, which, in turn, calls handleIncomingRedirect.
handleRedirectAfterLogin();
The handleIncomingRedirect() function collects the information provided by the Identity Provider. handleIncomingRedirect() is a no-op if called outside the login processs.
For more information on using the library to authenticate, see Authentication.
Get Pod(s) Code#
The example uses Inrupt’s solid-client
library to return the Pod(s)
associated with a WebID.
Import#
The application uses various objects from @inrupt/solid-client
.
Additional import objects are displayed for the other read and write
operations used in the application.
import {
addUrl,
addStringNoLocale,
createSolidDataset,
createThing,
getPodUrlAll,
getSolidDataset,
getThingAll,
getStringNoLocale,
removeThing,
saveSolidDatasetAt,
setThing
} from "@inrupt/solid-client";
import { SCHEMA_INRUPT, RDF, AS } from "@inrupt/vocab-common-rdf";
Get Pods#
The application uses getPodUrlAll to retrieve the Pod URLs (i.e.,
the value stored under http://www.w3.org/ns/pim/space#storage
) in
the user’s profile.
const webID = document.getElementById("myWebID").value;
const mypods = await getPodUrlAll(webID, { fetch: fetch });
For more information on properties of
Things
, and see Structured Data (RDF Resources).For more information on read operations, see Read/Write Structured Data.
For more information on property identifiers and vocabularies, see Vocabulary.
Write Reading List#
Import#
The application uses various objects from solid-client
and
vocab-common-rdf
libraries to write data to your Pod. Additional
import objects are shown for read profile operations.
import {
addUrl,
addStringNoLocale,
createSolidDataset,
createThing,
getPodUrlAll,
getSolidDataset,
getThingAll,
getStringNoLocale,
removeThing,
saveSolidDatasetAt,
setThing
} from "@inrupt/solid-client";
import { SCHEMA_INRUPT, RDF, AS } from "@inrupt/vocab-common-rdf";
Create Reading List SolidDataset#
The application uses getSolidDataset to retrieve an existing reading list from the URL.
If found, the application uses removeThing to clear the reading list by removing all titles from the list.
If not found (i.e., errors with 404 ), the application uses createSolidDataset to create a new SolidDataset (i.e., the reading list).
let myReadingList;
try {
// Attempt to retrieve the reading list in case it already exists.
myReadingList = await getSolidDataset(readingListUrl, { fetch: fetch });
// Clear the list to override the whole list
let items = getThingAll(myReadingList);
items.forEach((item) => {
myReadingList = removeThing(myReadingList, item);
});
} catch (error) {
if (typeof error.statusCode === "number" && error.statusCode === 404) {
// if not found, create a new SolidDataset (i.e., the reading list)
myReadingList = createSolidDataset();
} else {
console.error(error.message);
}
}
Tip
As an alternative to fetching an existing reading list and removing all titles from the list, you can instead attempt to deleteSolidDataset first, and then use createSolidDataset.
Add Items (Things) to Reading List#
For each title entered by the user:
The application uses createThing to create a new reading item (i.e., Thing). [2]
To the item, the application uses the following functions to add specific data:
addUrl to add the
http://www.w3.org/1999/02/22-rdf-syntax-ns#type
property with the URL valuehttps://www.w3.org/ns/activitystreams#Article
The example uses the
RDF.type
andAS.Article
convenience objects to specify the aforementioned property and value.addStringNoLocale to add the
https://schema.org/name
property with the string value set to one of the entered titles.The example uses the
SCHEMA_INRUPT.name
convenience object for the aforementioned property.
Then, the application uses setThing to add the item to the SolidDataset (i.e., the reading list).
let i = 0;
titles.forEach((title) => {
if (title.trim() !== "") {
let item = createThing({ name: "title" + i });
item = addUrl(item, RDF.type, AS.Article);
item = addStringNoLocale(item, SCHEMA_INRUPT.name, title);
myReadingList = setThing(myReadingList, item);
i++;
}
});
The solid-client
library’s functions (such as the various
add/set functions) do not modify the objects that are passed in
as arguments. Instead, the library’s functions return a new
object with the requested changes.
The application specifies the Thing’s name (optional) during its
instantiation. Typically, a Thing’s URL is its SolidDataset URL
(which ends with a /
) appended with #
and the Thing’s name;
in this case:
${podURL}getting-started/readingList/myList#title1
,${podURL}getting-started/readingList/myList#title2
, etc.
Save Reading List (SolidDataset)#
Tip
For the sake of simplicity and brevity, this getting started guide hardcodes the SolidDataset URL. In practice, you should add a link to this resource in your profile that applications can follow.
Use saveSolidDatasetAt to
save the reading list to
<PodURL>getting-started/readingList/myList
.
saveSolidDatasetAt
creates any intermediate folders/containers as needed. [3]
let savedReadingList = await saveSolidDatasetAt(
readingListUrl,
myReadingList,
{ fetch: fetch }
);
Upon successful save, saveSolidDatasetAt returns a SolidDataset whose state reflects the data that was sent to be saved.
See also Create vs. Update Operations.
The solid-client
library also provides the
saveSolidDatasetInContainer.
However, unlike saveSolidDatasetAt
which creates any intermediate folders/containers as needed,
saveSolidDatasetInContainer
requires that the specified destination container already exists.
Verify the Save Operation#
The save operation returns the SolidDataset (the reading
list) whose state reflect the data that was sent to be saved. The
savedReadingList
may not accurately reflect the saved
state of the data if concurrent operations have modified
additional fields.
To ensure you have the latest data, the tutorial uses getSolidDataset again after saving the data.
savedReadingList = await getSolidDataset(readingListUrl, { fetch: fetch });
let items = getThingAll(savedReadingList);
let listcontent = "";
for (let i = 0; i < items.length; i++) {
let item = getStringNoLocale(items[i], SCHEMA_INRUPT.name);
if (item !== null) {
listcontent += item + "\n";
}
}
document.getElementById("savedtitles").value = listcontent;
The application uses SCHEMA_INRUPT.name
convenience object
from the vocab-common-rdf
library to specify the property to
retrieve.